JavaScript is a powerful scripting language that provides developers with the flexibility they are looking for. Among all the features it offers arrays are best categorized as basic data types. These arrays become even more destructive when the power of higher order functions is invoked. In this guide, you’ll learn about the higher order array functions in Javascript and how you can take your code to the next level of efficiency and cleanliness.
Table of Contents
What Are Higher Order Functions?
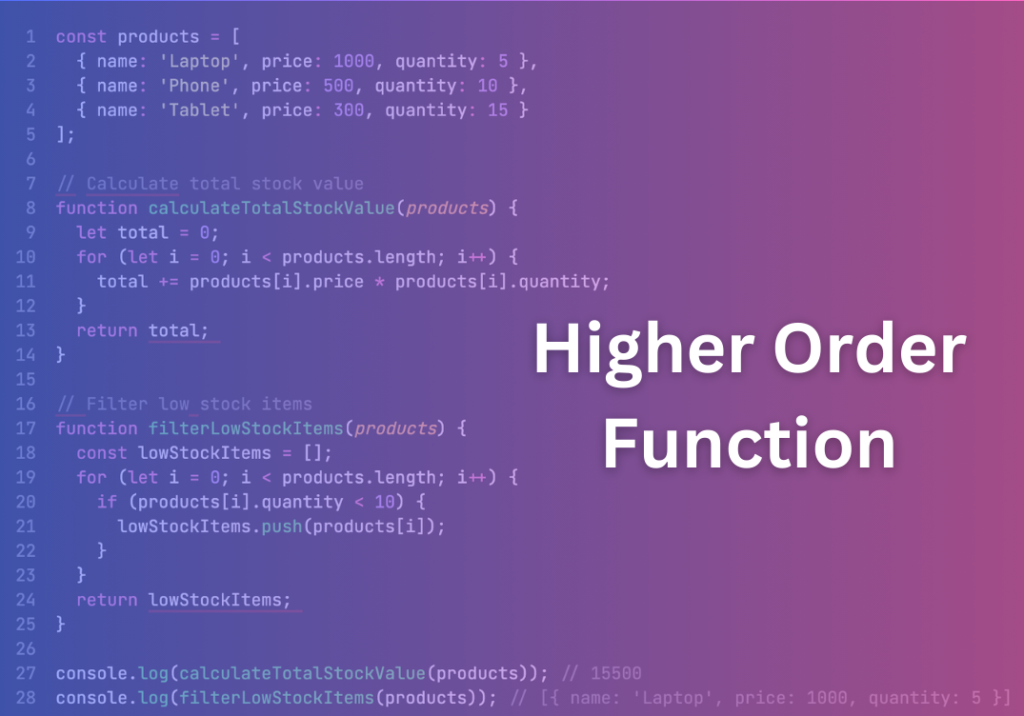
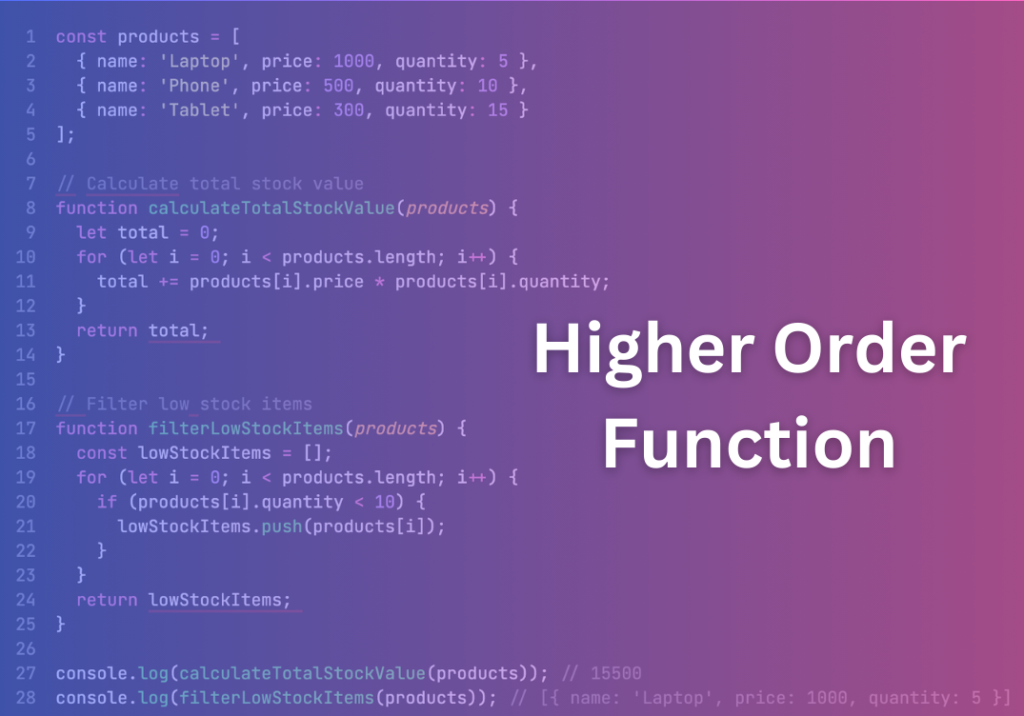
Higher order functions play a great role in JavaScript or let’s better say that they revolutionized it. They either accept other functions as their parameters or the result of the function is a function. This concept would make the code more versatile and could be utilized time and again. With arrays, simple operations such as transform, filter and aggregate can be achieved through higher order functions.
Here’s a basic example to illustrate what a higher order function is:
// A simple callback function
function notify() {
console.log('Notification sent!');
}
// A higher-order function that takes another function as an argument
function processTransaction(transaction, callback) {
console.log(`Processing transaction for ${transaction.amount} USD`);
callback(); // Executing the callback function
}
const transaction = { amount: 100 };
processTransaction(transaction, notify);
In this example, processTransaction
is a higher order function because it accepts another function (notify
) as an argument.
How Higher Order Functions Work
The functions carry out more functionalities because JavaScript supports first-order functions, which are functions that can be passed as parameters and returned as values within other functions. This capability enables the creation of generalized code to support general entities.
Let’s create an example: Managing Inventory
Let’s say we need to manage an inventory of products in a store. We might want to perform different operations like calculating total stock value, filtering low stock items, and applying discounts.
Without Higher Order Functions
const products = [
{ name: 'Laptop', price: 1000, quantity: 5 },
{ name: 'Phone', price: 500, quantity: 10 },
{ name: 'Tablet', price: 300, quantity: 15 }
];
// Calculate total stock value
function calculateTotalStockValue(products) {
let total = 0;
for (let i = 0; i < products.length; i++) {
total += products[i].price * products[i].quantity;
}
return total;
}
// Filter low stock items
function filterLowStockItems(products) {
const lowStockItems = [];
for (let i = 0; i < products.length; i++) {
if (products[i].quantity < 10) {
lowStockItems.push(products[i]);
}
}
return lowStockItems;
}
console.log(calculateTotalStockValue(products)); // 15500
console.log(filterLowStockItems(products)); // [{ name: 'Laptop', price: 1000, quantity: 5 }]
With Higher Order Functions
const products = [
{ name: 'Laptop', price: 1000, quantity: 5 },
{ name: 'Phone', price: 500, quantity: 10 },
{ name: 'Tablet', price: 300, quantity: 15 }
];
// Higher order function to perform operations on products
function processProducts(products, operation) {
return products.map(operation);
}
// Operations
function calculateStockValue(product) {
return product.price * product.quantity;
}
function checkLowStock(product) {
return product.quantity < 10;
}
// Using the higher order function
const stockValues = processProducts(products, calculateStockValue);
const lowStockItems = products.filter(checkLowStock);
console.log(stockValues); // [5000, 5000, 4500]
console.log(lowStockItems); // [{ name: 'Laptop', price: 1000, quantity: 5 }]
By using processProducts
as a higher order function, we avoid code repetition and make our code more modular and easier to maintain.
Common Higher Order Array Functions
Let’s delve into some commonly used higher order array functions in JavaScript: forEach
, map
, filter
, reduce
, and find
.
forEach
The forEach function allows you to run a function on each element of an array. It’s primarily used for side effects like logging or modifying elements.
const orders = [
{ id: 1, amount: 250 },
{ id: 2, amount: 400 },
{ id: 3, amount: 150 }
];
orders.forEach(order => {
console.log(`Order ID: ${order.id}, Amount: ${order.amount}`);
});
map
The map function is used to transform each element in an array and create a new array with the transformed elements.
const salaries = [3000, 5000, 7000];
const increasedSalaries = salaries.map(salary => salary * 1.1);
console.log(increasedSalaries); // [3300, 5500, 7700]
filter
The filter function creates a new array with elements that pass a test provided by a function.
const employees = [
{ name: 'Alice', age: 25 },
{ name: 'Bob', age: 35 },
{ name: 'Charlie', age: 30 }
];
const youngEmployees = employees.filter(employee => employee.age < 30);
console.log(youngEmployees); // [{ name: 'Alice', age: 25 }]
reduce
The reduce function reduces an array to a single value by applying a function to each element and an accumulator.
const expenses = [200, 300, 150];
const totalExpenses = expenses.reduce((acc, expense) => acc + expense, 0);
console.log(totalExpenses); // 650
find
The find function returns the first element that satisfies a condition provided by a function. If no element passes the test, it returns undefined
.
const customers = [
{ name: 'Dave', purchaseAmount: 1200 },
{ name: 'Eve', purchaseAmount: 800 },
{ name: 'Frank', purchaseAmount: 1500 }
];
const highSpender = customers.find(customer => customer.purchaseAmount > 1000);
console.log(highSpender); // { name: 'Dave', purchaseAmount: 1200 }
Practical Real-World Examples
To make the concept of higher order functions even clearer, let’s explore a few more real-world scenarios.
Processing User Data
Imagine you have an array of user objects, and you need to perform various operations such as finding users from a specific city, calculating the average age, and extracting emails.
const users = [
{ name: 'A', age: 28, city: 'New York', email: 'a@example.com' },
{ name: 'B', age: 22, city: 'Los Angeles', email: 'b@example.com' },
{ name: 'C', age: 32, city: 'New York', email: 'c@example.com' },
{ name: 'D', age: 24, city: 'Chicago', email: 'd@example.com' }
];
// Finding users from New York
const newYorkUsers = users.filter(user => user.city === 'New York');
console.log(newYorkUsers); // [{ name: 'A', age: 28, city: 'New York', email: 'a@example.com' }, { name: 'C', age: 32, city: 'New York', email: 'c@example.com' }]
// Calculating average age
const totalAge = users.reduce((acc, user) => acc + user.age, 0);
const averageAge = totalAge / users.length;
console.log(averageAge); // 26.5
// Extracting emails
const emails = users.map(user => user.email);
console.log(emails); // ['a@example.com', 'b@example.com', 'c@example.com', 'd@example.com']
Analyzing Sales Data
Suppose you have an array of sales records, and you need to perform tasks such as calculating total revenue, finding high-value sales, and generating a list of customer names.
const sales = [
{ customer: 'Alice', amount: 300, region: 'North' },
{ customer: 'Bob', amount: 700, region: 'South' },
{ customer: 'Charlie', amount: 150, region: 'North' },
{ customer: 'Dave', amount: 800, region: 'South' }
];
// Calculating total revenue
const totalRevenue = sales.reduce((acc, sale) => acc + sale.amount, 0);
console.log(totalRevenue); // 1950
// Finding high-value sales (amount > 500)
const highValueSales = sales.filter(sale => sale.amount > 500);
console.log(highValueSales); // [{ customer: 'Bob', amount: 700, region: 'South' }, { customer: 'Dave', amount: 800, region: 'South' }]
// Generating a list of customer names
const customerNames = sales.map(sale => sale.customer);
console.log(customerNames); // ['Alice', 'Bob', 'Charlie', 'Dave']
Advanced Use Case: Composing Functions for Data Transformation
You can also use higher order functions to create more complex operations by composing functions. This is especially useful for data transformation pipelines.
const numbers = [1, 2, 3, 4, 5];
// Functions to double and square numbers
const double = x => x * 2;
const square = x => x * x;
// Composing functions
const compose = (f, g) => x => f(g(x));
const doubleAndSquare = compose(square, double);
const results = numbers.map(doubleAndSquare);
console.log(results); // [4, 16, 36, 64, 100]
In this code, compose
is a higher order function that takes two functions and returns a new function that is their composition.
Advanced Use Case: Composing Functions
You can also use higher order functions to create more complex operations by composing functions. This is especially useful for data transformation pipelines.
const numbers = [1, 2, 3, 4, 5];
// Functions to double and square numbers
const double = x => x * 2;
const square = x => x * x;
// Composing functions
const compose = (f, g) => x => f(g(x));
const doubleAndSquare = compose(square, double);
const results = numbers.map(doubleAndSquare);
console.log(results); // [4, 16, 36, 64, 100]
In this example, compose
is a higher order function that takes two functions and returns a new function that is their composition.
Benefits of Higher Order Functions
- Readability: Higher order functions make your code more concise and easier to understand.
- Reusability: They help you avoid code repetition by allowing you to write reusable functions.
- Maintainability: They make your code modular, which is easier to maintain and extend.
Conclusion
Higher order array functions in JavaScript are essential tools for any developer. They help you manipulate arrays more efficiently and write clearer code. Whether you’re transforming, filtering, or reducing data, these functions make your life easier. Start using them today to see the difference in your coding!
By understanding and using these higher order functions, you can significantly improve your JavaScript skills. Happy coding!
You May Also Like