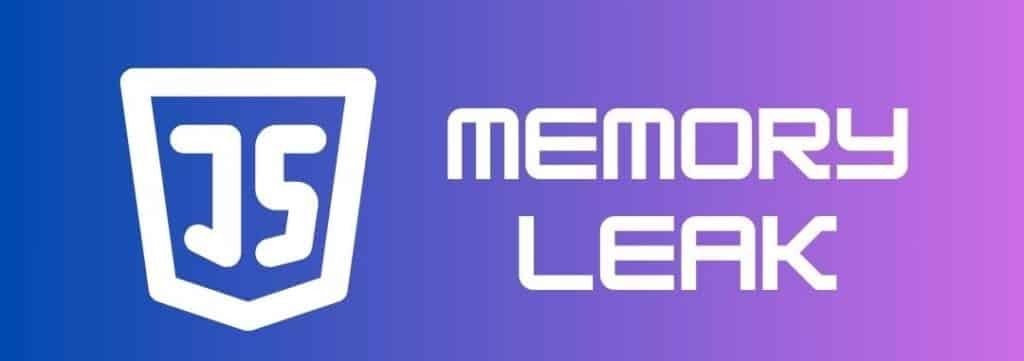
Top 7 Cause of JavaScript Memory Leaks and Prevention
Memory management is an essential factor in all programming languages, and JavaScript is no exception. JavaScript memory leaks can still occur despite advanced garbage collection techniques employed by JavaScript engines like V8 used in Chrome and Node.js. Consequently, such leakages can raise memory usage, impair performance and prompt application crashes.
In accordance with a report published in the “International Journal of Open Information Technologies” this has seen JavaScript evolve into an immensely complex language that is integrated in web portals, online games, media management and data science among other applications.
This upshot necessitates smart memory management because mistakes here cause memory leaks that compromise performance. Memory leaks usually result from unreleased memory which is often caused by circular references, closures or timers.
In spite of the availability of garbage collector in JavaScript for managing unreachable objects, developers must remain watchful. Google’s Chrome Developer Tools and Mozilla’s Developer Tools are examples of such tools that can be used to detect and profile these leaks leading to robust application performance.
Here we are going to discuss what memory leaks are, their common causes, how you can find them, and prevention practices with practical examples.
Table of Contents
What is a memory leak?
This happens when a program keeps references to objects that it does not need any more. Hence, the garbage collector cannot recover that memory. Over time, this leads to increased memory usage, which eventually exhausts available memory, causing performance degradation or crashes.
Causes of JavaScript Memory Leaks
1. Unintentional Global Variables
These variables are always reachable within the global scope, and therefore, it does not consider them eligible for garbage collection by default. Unintentionally creating global variables can cause memory leaks.
function createLeak() {
leak = 'This is a memory leak'; // 'leak' is a global variable
}
createLeak();
In the above example, you can see that a variable leak has been created without defining let, const, or var keyword, in this way, it is a global variable. Since this variable can still be accessed within the global scope, it won’t be garbage collected.
2. Forgotten Timers and Intervals
Timers as well as intervals may prevent variables from being collected by holding any references to them.
function createLeak() {
let element = document.getElementById('leakElement');
setInterval(() => {
console.log(element.innerText);
}, 1000);
}
createLeak();
Now you can see that the setInterval function creates a memory leak because it maintains a reference to the element
variable. Garbage collection is prevented because the interval retains the reference even after the element is deleted from the DOM.
3. Closures
Closures may retain references to outer function variables, leading to a case whereby they will not be freed up, thus causing memory leakage if not properly handled.
function createLeak() {
let largeArray = new Array(1000000).fill('*');
return function() {
console.log(largeArray.length);
};
}
let leakFunction = createLeak();
In this example, even though the reference to largeArray is no longer required after the function is formed, it is kept in the inner function. This causes a memory leak by preventing the largeArray from being garbage collected.
4. DOM Nodes without Connections
Detached DOM nodes are nodes that have been removed from the DOM, but can still be accessed via JavaScript and hence cannot be garbage collected.
function createLeak() {
let element = document.getElementById('leakElement');
document.body.removeChild(element);
// 'element' is still referenced
}
createLeak();
In this case, an element is taken out of the DOM even if a reference to it is kept in a variable named element. As a result, the memory used by the unattached DOM node will not be released by the garbage collector.
5. Events Listeners
Memory leaks can result from adding event listeners to DOM elements but not removing them especially when these elements are detached from the current document tree.
function createLeak() {
let element = document.getElementById('leakElement');
element.addEventListener('click', () => {
console.log('Element clicked');
});
// Removing element from DOM
document.body.removeChild(element);
}
createLeak();
For example, after removing the element from the DOM, a reference to it must still remain in an event listener on element so that there is no need for it to go through garbage collection process.
6. Outdated References in Data Structures
When referencing objects such as arrays or objects within data structures like arrays or objects , they may congest memory if they are left uncleared when no longer of any use.
let cache = {};
function createLeak() {
let element = document.getElementById('leakElement');
cache['leakedElement'] = element;
// Removing element from DOM
document.body.removeChild(element);
}
createLeak();
This time however has stored its reference in cache object whereby it cannot let go even though it has been removed from the parent and thus can not be gathered at all by any mean which means that it will remain as part of garbage-collectible object space.
7. Circular References
Circular references occur when multiple objects refer each other creating loops—this prevents cleanup.
function createLeak() {
let obj1 = {};
let obj2 = {};
obj1.reference = obj2;
obj2.reference = obj1;
}
createLeak();
Interaction with obj1 and obj2 leads to a circular reference. Hence, garbage collection may be blocked if the cycle is not interrupted.
Preventing Memory Leaks
1. Avoid Unintentional Global Variables
Create variables with let and const in order to avoid accidental creation of global variables.
function createLeak() {
let leak = 'This is no longer a memory leak'; // 'leak' is scoped to the function
}
createLeak();
The declaration of the leak variable as let confines it within the createLeak function thereby ensuring that it does not become a global variable which may remain uncollected even after the execution of the function.
2. Clear Timers and Intervals
All timers or intervals must be cleared when they are no longer needed.
function createLeak() {
let element = document.getElementById('leakElement');
let intervalId = setInterval(() => {
console.log(element.innerText);
}, 1000);
// Clear the interval when done
setTimeout(() => clearInterval(intervalId), 5000);
}
createLeak();
In this case, clearInterval is called on ‘interval’ after every five seconds thus ensuring the release of element for garbage collection purposes where it may become useless in future.
3. Manage Closure
Mind what data your closures capture and do not capture needless information.
function createLeak() {
let largeArray = new Array(1000000).fill('*');
return function() {
console.log('Closure executed');
};
}
let leakFunction = createLeak();
By modifying inner function so that largeArray is ignored, we prevent closure capturing unnecessary data hence decreasing probability of memory leakages.
4. Remove References to Detached DOM Nodes
References pointing to DOM nodes that have been removed from document should be set to null before such references are destroyed by JavaScript garbage collector.
function createLeak() {
let element = document.getElementById('leakElement');
document.body.removeChild(element);
element = null; // Remove reference
}
createLeak();
In this example, setting element to null after removing it from the DOM ensures that there are no lingering references, allowing the garbage collector to reclaim the memory used by the detached DOM node.
5. Unbind Event Listeners
Eradicate memory leaks by removing an event listener when it is no longer needed.
function createLeak() {
let element = document.getElementById('leakElement');
const handleClick = () => {
console.log('Element clicked');
};
element.addEventListener('click', handleClick);
// Removing element from DOM and listener
document.body.removeChild(element);
element.removeEventListener('click', handleClick);
element = null; // Clear reference
}
createLeak();
By taking away the event listener before or after deleting the DOM element, we make sure that there are no lingering references that can stop garbage collection.
6. Clear Outdated References in Data Structures
Prevent memory leaks by eliminating old data structure references.
let cache = {};
function createLeak() {
let element = document.getElementById('leakElement');
cache['leakedElement'] = element;
// Removing element from DOM and cache
document.body.removeChild(element);
delete cache['leakedElement'];
element = null; // Clear reference
}
createLeak();
When we remove the reference from cache object and set element to null, then we have enabled the garbage collector to get back this memory.
7. Break Circular References
To avoid memory leaks, break circular references.
function createLeak() {
let obj1 = {};
let obj2 = {};
obj1.reference = obj2;
obj2.reference = obj1;
// Breaking the circular reference
obj1.reference = null;
obj2.reference = null;
}
createLeak();
Thus nullifying references is what breaks the circle ensuring that the garbage collector frees up space for other uses as well as freeing up space previously circled off.
Detecting Memory Leaks
Using Chrome DevTools
Chrome DevTools provides powerful tools for detecting memory leaks.
- Open DevTools: Right-click on your webpage and select “Inspect” or press
Ctrl+Shift+I
(Windows/Linux) orCmd+Opt+I
(Mac). - Go to the Memory Tab: Click on the “Memory” tab.
- Take a Heap Snapshot: Click the “Take snapshot” button to capture the current memory state.
- Analyze the Snapshot: Look for objects that should have been garbage collected but are still present.
Using Performance Profiling
- Go to the Performance Tab: Click on the “Performance” tab in DevTools.
- Start Recording: Click the “Record” button and interact with your webpage to simulate normal usage.
- Stop Recording: Click the “Stop” button after a period of time.
- Analyze the Profile: Look for memory usage trends and identify any unexpected increases.
Conclusion
Understanding common causes of, and how to detect them will make you write a more efficient and reliable JavaScript code whereas they may not be easily spotted thus requiring some understanding on their nature. In this regard, best practices such as avoiding accidental global variables, clearing timers, handling closures properly and releasing any detached reference to DOM elements like event listeners, data structures and circular references can mitigate us against risks associated with these symptoms of memory leakages in our applications.
Keep in mind that preemptive retention management helps achieve optimal performance today thereby preventing future problems. Good luck with programming!
References:
- MDN Web Docs: Memory Management
- Google Chrome Developers: Memory Leaks
- JavaScript: The Good Parts by Douglas Crockford
You May Also Like